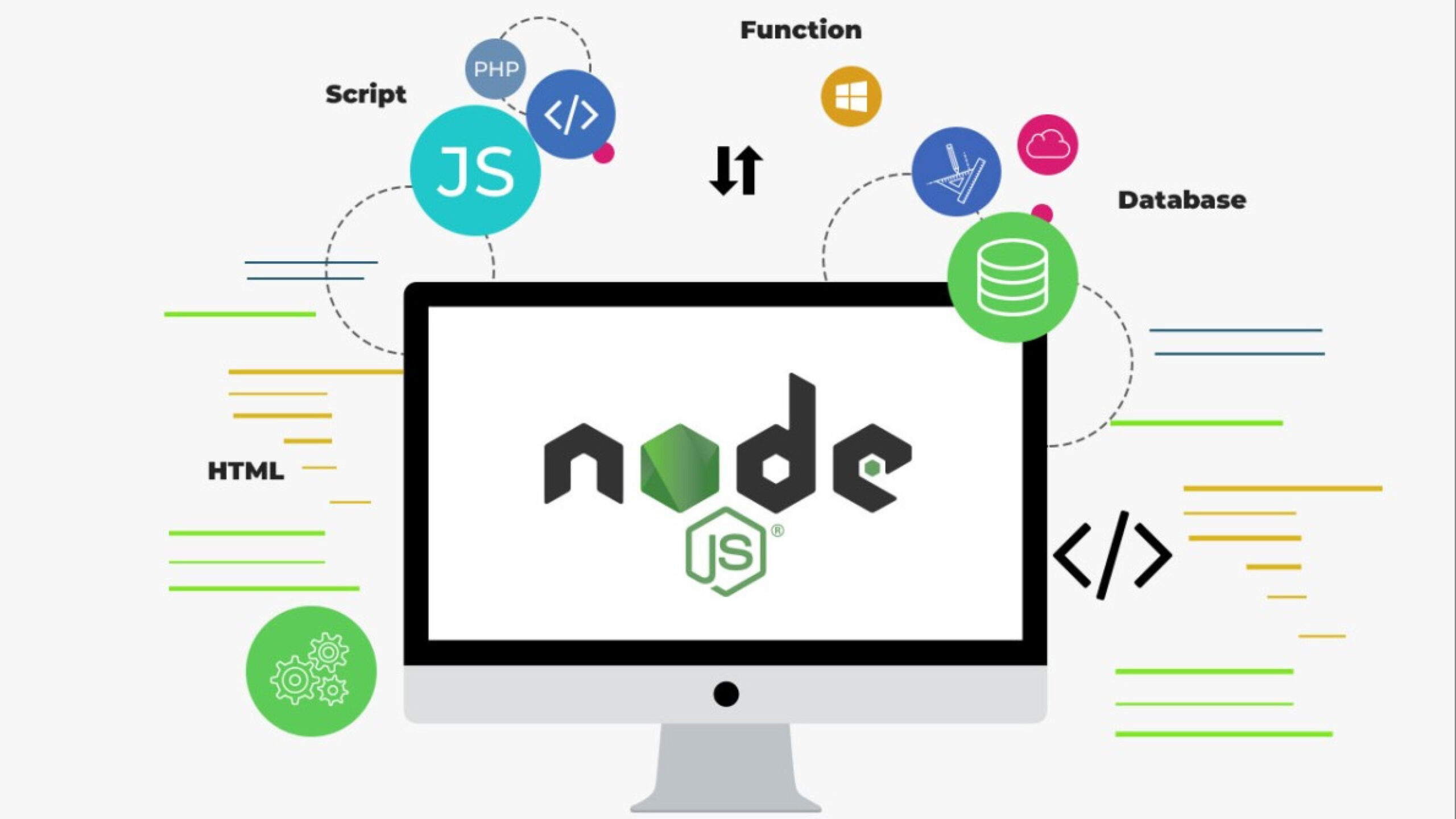
Best Practices For Developing Applications With Node.js Architecture
A leading streaming service, Netflix encountered several challenges with its software framework that hindered business growth. The monolithic architecture proved to be a hindrance to scaling, and the heavy-weight back-end of JAVA made development and deployment slow. Additionally, there were difficulties with the front-end and back-end transition, which resulted in longer load times and increased latency. To overcome these challenges, Netflix adopted Node.js. This led to a significant reduction in startup loading time by 70%.
The transition between the front-end and back-end also improved significantly, resulting in reduced latency. Node.js, being asynchronous and non-blocking, made scaling much more accessible, enabling the service to handle millions of requests simultaneously. Other companies such as NASA, Trello, PayPal, Uber, and Twitter have also experienced similar benefits from adopting Node.js. However, to achieve these results, it’s essential to have a thorough understanding of Node.js Architecture and best practices for application development. This article aims to provide you with all the necessary information on Node.js Architecture and best practices for Node.js application development.
Understanding Node.js Architecture
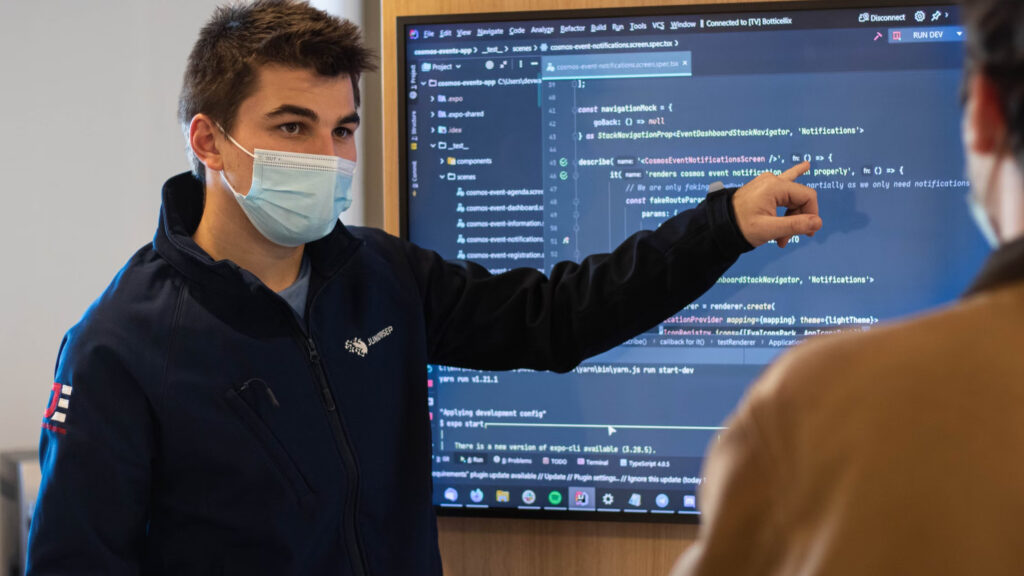
Node.js uses a “Single Threaded Event Loop” architecture to handle multiple concurrent clients. The Node.js processing model is built on a Javascript-event-based model and a callback mechanism. This event loop-based mechanism allows Node.js to run blocking I/O operations in a non-blocking way. Additionally, scaling is much simpler with a single thread than using one line or a new thread per request under typical web loads.
Now, let’s dive deeper into the key elements that make up the Node.js Architecture.
Critical Elements Of Node.js Architecture
Node.js Architecture comprises several vital elements that handle client requests and provide results. These elements include:
Requests: Depending on the specific tasks users need to perform in a web application, requests can be classified as either blocking (complex) or non-blocking (simple).
Node.js Server: This is the core component of the architecture that accepts user requests, processes them, and returns the results to the corresponding users.
Event Queue: It stores the incoming requests and passes them sequentially to the Event Pool.
Event Pool: After receiving client requests from the event queue, it sends responses to the corresponding clients.
Thread Pool: It contains the threads available for performing the operations necessary to process requests.
External Resources: These resources are used for blocking client requests and can be used for computation, data storage, and more.
Advantages Of Node.js Architecture
Node.js Architecture offers several benefits that make it the right choice for building applications. Some of the key advantages are:
Scalability
The non-blocking I/O model and the system kernel’s worker pool allow for building highly scalable Node.js applications. These apps can handle thousands of concurrent client requests without overwhelming the system. Additionally, Node.js’ lightweight nature enables leveraging microservices architecture. Walmart, for example, implemented the microservices architecture with Node.js and saw a 20% conversion growth, 100% uptime on Black Friday sales handling over 500 million page views and saving up to 40% on hardware.
Speed And Performance
The event-driven programming of Node.js is one of the main reasons for its higher speed compared to other technologies. It helps in synchronizing the occurrence of multiple events and building a simple program. Moreover, its non-blocking input-output operations contribute to its speed. As the code runs faster, it improves the entire run-time environment.
Flexibility
Node.js allows for making changes in specific Nodes rather than requiring changes to the core programming as in other frameworks. This feature benefits the initial build stage and ongoing maintenance.
However, to reap these benefits, following best practices for Node.js application development is essential.
Best Practices For Node.js Application Architecture

To ensure that your Node.js application is efficient, scalable, and easy to maintain, it’s essential to follow best practices for Node.js application architecture. Some of the crucial best practices include:
Incorporate The Publisher-Subscriber Model
The publisher-subscriber model is a data exchange pattern that includes two communicating systems: publishers and subscribers. Publishers must learn about the receiving system to send messages through specific channels. Subscribers receive and deal with one or more of these channels without the publishers’ ability. Adopting this model allows you to manage multiple child operations connected to a single action.
For example, sending event notifications to a large user base can be challenging as you need systems to deliver the messages rapidly. However, as the user base increases, ensuring that all clients receive the message becomes challenging. The pub/sub model follows a loose coupling principle, allowing the publisher to send event notifications without worrying about the client’s status. The model also ensures robust implementation of brokers, which can persist messages and deliver them exactly once. So, publishers can simply “fire and forget,” while brokers provide the notice when clients need them.
Another use case of the pub/sub model is distributed caching. Caches play a significant role in improving the system’s performance. With the pub/sub model, you can implement distributed caching efficiently. You don’t need to wait for the client’s request to seed the cache. Instead, you can do that in advance asynchronously. This saves time and improves app performance.
Adopt A Layered Approach
A layered approach to Node.js application architecture can help developers follow the “separation of concerns” principle. The popular Node.js framework Express.js allows developers to divide the codebase into business logic, database, and API routes. These fall into three different layers: service layer, controller layer, and data access layer. The idea is to separate the business logic from Node.js API routes to avoid complex background processes.
Controller Layer
This layer defines the API routes. The route handler functions allow you to deconstruct the request object, collect the necessary data pieces, and pass them on to the service layer for further processing.
Service Layer
This layer is responsible for executing the business logic. It includes classes and methods that follow S.O.L.I.D programming principles, perform singular responsibilities, and are reusable. The coating also decouples the processing logic from the defining point of routes.
Data Access Layer
This layer is responsible for handling the database. It fetches from, writes to, and updates the database. It also defines SQL queries, database connections, models, ORMs, etc. The three-layered setup acts as a reliable arrangement for Node.js applications. These stages make your application easier to code, maintain, debug and test.
Use Dependency Injection
Dependency injection is a software design pattern that advocates passing (injecting) dependencies (or services) as parameters to modules instead of requiring or creating specific ones inside them. This pattern keeps modules more flexible, independent, reusable, scalable, and easily testable across the application.
Leverage Third-Party Solutions
Node.js has a large developer community and offers a powerful package manager, NPM, filled with feature-rich, well-maintained, well-documented frameworks, libraries, and tools. This makes it easy for developers to incorporate these existing solutions into their code and take advantage of their APIs.
Some useful Node.js libraries to consider
- Moment for working with dates and times
- Nodemon for automatically restarting the app when code updates
- Agenda for job scheduling
- Winston for logging
- Grunt for automating tasks
When using third-party solutions, it’s essential to understand their purpose, benefits, and dependencies. Careful consideration should be given before importing them, and it’s necessary to rely on something other than third-party solutions as they can introduce security risks or too many dependencies.
Implement A Consistent Folder Structure
We previously discussed the importance of adopting a layered approach for Node.js applications. A consistent folder structure can bring this approach to life by organizing different modules into separate folders. This organization clarifies which functionalities, classes, and methods are used in the application.
Ensure Clean Coding With Linters, Formatters, Style Guides, And Comments
To ensure that your Node.js application code is clean, readable, and maintainable, it’s essential to use linters, formatters, style guides, and comments.
Linting And Formatting
Code linters are static code analysis tools that automatically check for programming errors, styles, bugs, and suspicious constructs. Formatters ensure consistent formatting and styling across your entire project. Popular linter tools for inspecting Javascript code include JSLint and ESLint, while Prettier is a popular code formatting tool. Many modern IDEs or code editors also provide linters and formatters as plugins, along with other valuable features such as intelligent code completion, auto imports, on-hover documentation support, debugging tools, code navigation, and refactorings.
Style Guides
To improve code readability and consistency, consider adopting a style guide like those provided by Google or Airbnb for Javascript. These guides cover everything from naming conventions and formatting to file system encodings. Using style guides can also help you follow the standards used by some of the top developers across the globe.
Adding Comments
Adding comments to your code is an effective way to enhance readability and understanding of the purpose of a particular code. Comments can also help when revisiting the code after some time. Additionally, comments are an excellent way to document your project details, such as APIs, classes, functions, author name, version, etc.
Rectify Errors With Unit Testing, Logging, And Error-Handling
To ensure that your Node.js application is functioning correctly, it’s essential to use unit testing, logging, and error handling.
Unit Testing
Unit testing helps to improve code quality and identify problems early on by isolating a section of code and verifying its accuracy, validity, and robustness. It can be performed in Node.js applications using test frameworks such as Jest, Mocha, or Jasmine.
Logging And Error-Handling
Errors are an inevitable part of programming, and it is vital to have a way to handle and log them. Node.js has built-in logging systems that log essential information throughout the development process and make debugging easier. The most common form of logging information in Node.js is the console.log() method. Logging information in Node.js checks through three streams: stdin, stdout, and stderr. stdin deals with input to the process, stdout deals with the output to the console or a separate file, and stderr deals specifically with error messages and warnings.
To avoid callback issues and simplify the error-handling process in Node.js, creating a centralized error-handling component is recommended. This component sends error messages to system admins, transfers events to monitoring services, and logs every event.
Practice Writing Asynchronous Code
Asynchronous code execution is an essential aspect of Node.js development. Callback functions have traditionally been used to define asynchronous behaviour. Still, as the number of chained operations increases, the code can become more complex and challenging to handle, leading to a “callback hell.”
To avoid this issue, it’s essential to practice asynchronous writing code using modern techniques such as Promises and async/awaits.
Using Config File And Environment Variables
As an application grows, it’s essential to have global configuration options that every module can access. A separate file can store these options in a config folder within the project structure. These options may include basic settings, API keys, database connections, etc. It’s recommended to store these options as environment variables in a .env file in the form of key-value pairs.
To access these environment variables in your code, you can use an NPM package like dotenv. It’s a best practice to import environment variables into one central place so that any changes will be reflected throughout the application.
Employ Gzip Compression
Gzip is a lossless compression mechanism used to compress files for faster transfer. Node.js is often used for heavy-weight web development pages containing multimedia files, so Gzip compression can help reduce load and improve speed. Express.js, a popular Node.js framework, includes built-in support for Gzip compression through compression middleware. Many Express.js documentation recommends the use of Gzip compression to improve performance.
Use APM Tools
For enterprise-level applications developed with Node.js, ensuring a world-class user experience and high performance is crucial. This is where APM (Application Performance Monitoring) tools come into play. These tools help to monitor the app performance continuously and detect errors or bottlenecks that may impact the user experience. They also provide real-time insights. By continuously monitoring and addressing various issues, there is a greater chance that your application will perform at its best for an extended period. Some popular Node.js monitoring tools include Appmetrics, Prometheus, and Express Status Monitor. In the case of SenTMap, a sentiment-based market analytics engine, our engineering team decided to build a robust back-end using Node.js. Due to the asynchronous and non-blocking nature of Node.js, the application could efficiently process large amounts of data in real time. Additionally, it helped SenTMap to reduce server RAM requirements and handle 1 million concurrent users.
Manish Surapaneni
A Visionary & Expert in enhancing customer experience design, build solutions, modernize applications and leverage technology with Data Analytics to create real value.
Other articles

Manish Surapaneni
Stop Outsourcing Your Ethics, Tech Leaders
Setting up their own ethics committee is a step forward for tech companies, as they are the clear winners of
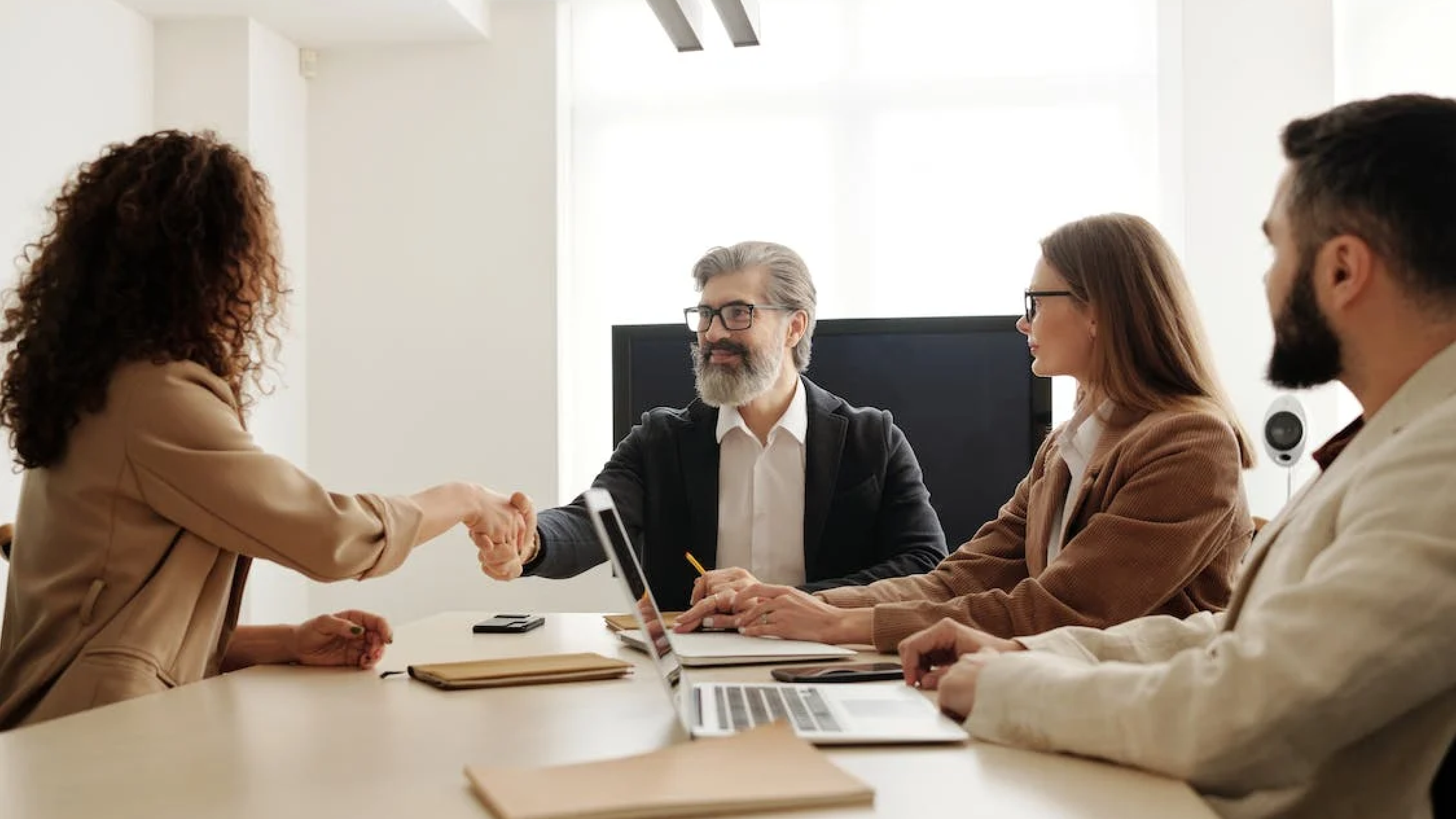
Manish Surapaneni
How To Beat The Tech Talent Squeeze In 2023: 6 Proven Strategies
In the current economic climate, with rising costs and increased job turnover, one of the biggest mistakes a company can

Manish Surapaneni
How Augmented Human Intelligence Can Enhance Our Lives With Machines
The advancement of technology has simplified our lives. It has also significantly increased our reliance on electronic devices. MIT Media