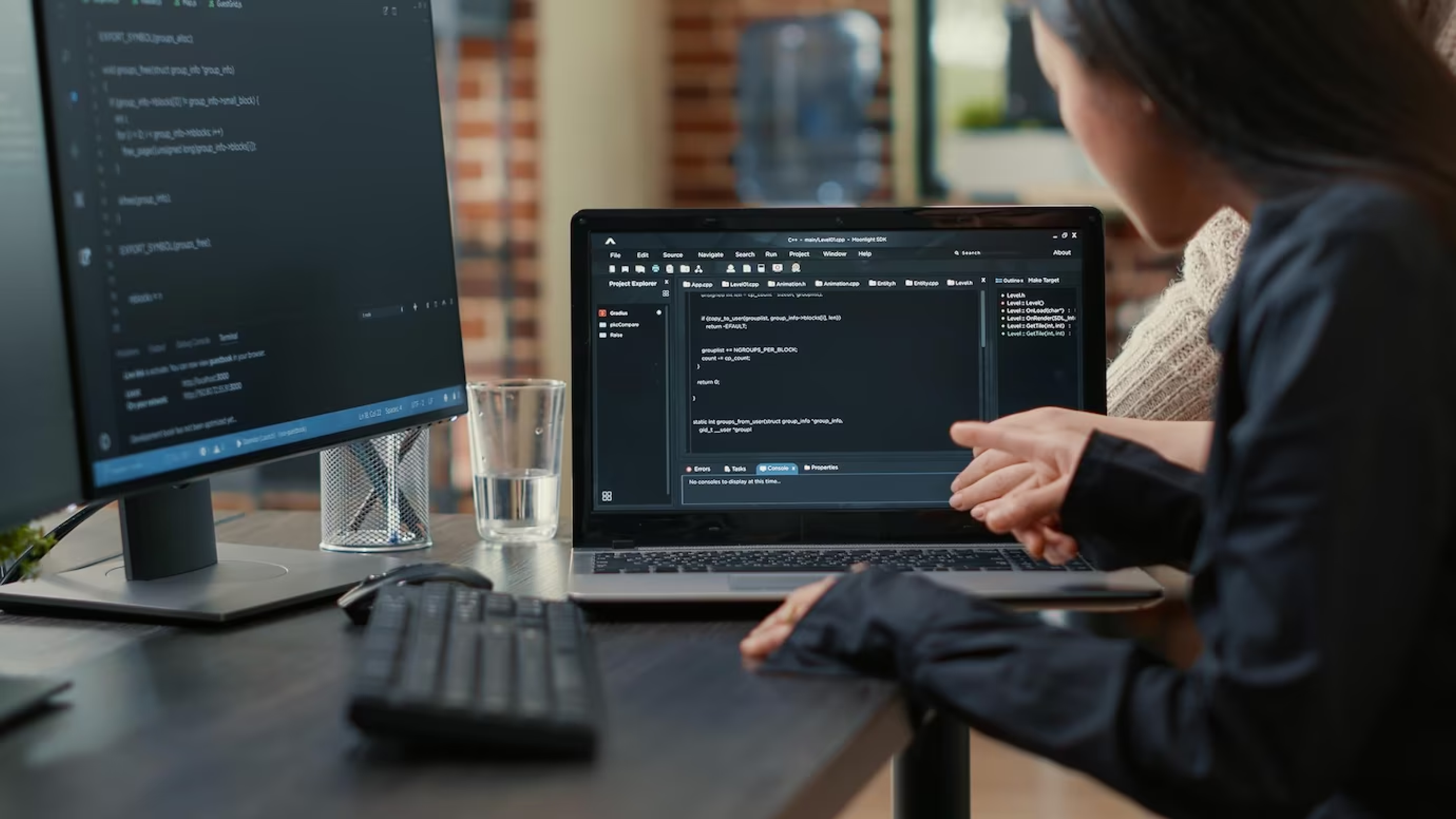
Practical Unit Testing: 10 Strategies For Maximizing Test Efficiency
When the Web Server team at Google implemented a culture of Unit Testing, they set a rule that no code or code review could be accepted without accompanying unit tests. This change significantly impacted their development process, resulting in faster development speeds, higher test coverage, and a substantial reduction in defects, production rollbacks, and emergency releases. Unit Testing also helped to discipline the entire team and provided concrete documentation for new team members. Unsurprisingly, many organizations now use Unit Testing to prevent bugs and improve software quality. However, it’s essential to approach Unit Testing correctly to realize its benefits fully. This blog will provide you with the best practices for integrating Unit Testing into your development and testing cycle to maximize the help of this essential testing strategy.
What Is Unit Testing?
Unit Testing tests individual units or components of software to ensure they function as intended. It involves breaking down code into small, testable units and testing them individually. This allows for more efficient and effective testing, allowing developers to locate and fix errors early in the development cycle quickly.
Unit Testing is a type of functional testing that software developers typically perform. The scope of what constitutes a “unit” can vary depending on the programming paradigm, with an entire class or interface being considered a unit in object-oriented programming and an individual function being considered a unit in procedural programming.
The ultimate goal of Unit Testing is to validate and compare the actual behaviour of the software with the expected behaviour. However, Unit Testing can be costly and require significant training, experience, and effort to build and maintain the tests. Additionally, it may only be suitable for some projects with short timelines.
Despite these challenges, Unit Testing can be a valuable tool for organizations when implemented correctly. To ensure the success of Unit Testing in your software development life cycle, it’s essential to understand its role and to match your unit tests with the appropriate characteristics.
The Role Of Unit Testing
Unit Testing plays a vital role in the software development life cycle (SDLC) by providing early, rapid, and continuous feedback. It offers precise input by focusing on individual code units and helps to ensure that quality standards are met before deploying the product.
Software engineers typically create Unit Testing to verify how units work when they are entirely isolated. Developers use test doubles such as stubs and mock objects to replace missing parts in a test module for remote testing.
Unit Testing enables the continuous testing of app modules without needing external services or dependencies. Overall, it provides developers with a reliable engineering environment where efficiency, productivity, and quality code are paramount.
Characteristics Of A Good Unit Test
Unit Testing is an essential part of the software development process, and it is crucial to understand what makes a good unit test. The following are the characteristics of a good unit test:
- Fast: Unit tests should dash, as a project can have thousands of unit tests. Slow-running unit tests can frustrate testers and take a long time to execute. Also, unit tests are run repeatedly, so developers might skip running them and deliver buggy code if they are slow.
- Reliable: Unit tests should only fail if there is a bug in the underlying code. However, sometimes tests fail even if there are no bugs in the source code due to a design flaw. For example, a test may pass one by one, but it may fail when executing the whole test class or on the CI server. Additionally, unit tests should be reusable and help avoid repetitive work.
- Isolated: Unit tests should be run in isolation without interdependencies, external dependencies (file system, database, etc.) or external environmental factors.
- Self-checking: Unit tests should automatically determine whether they passed or failed without human intervention.
- Timely: Unit test code should not take long to write than tested code. A good unit test is easy to write and is not tightly coupled.
- Truly unit, not integration: Unit tests should be standalone and not be influenced by external factors. They should not turn into integration tests if tested with multiple other components.
Maintaining standard practices for software testing, including unit tests, is essential. The testing guidelines can vary depending on the platform and project, but it’s possible to stick to a general standard of best practices to unit test the product.
Unit Testing Best Practices

Unit Testing is an important aspect of software development, and it is essential to follow best practices to ensure that tests are practical and efficient. The following are some best practices to keep in mind when writing unit tests:
1. Write Readable Tests
Easy-to-read tests are essential for understanding how code works, its intent, and what goes wrong when the test fails. Tests that reveal the setup logic at first glance are more convenient for figuring out how to fix the problem without debugging the code. Such readability also improves the maintainability of tests since the production code changes are also required to be updated in the tests. Moreover, difficult-to-read tests create more misunderstandings among developers, resulting in more bugs.
Unit tests also serve as documentation, as they describe the behavioural aspect of the subject and validate it. So when you write clear and readable tests, you’re not only doing your future self a favour but also other new developers on the team or still need to be hired. It instantly familiarizes them with the code and entire systems without bothering anyone else.
Ways to write accessible and enjoyable tests to read:
- Have a good naming convention for every test case. Name tests in such a way that it instantly describes the subject, the scenario being tested, and the expected result.
- Use the Arrange, Act, Assert pattern to define the test phases and enhance readability.
- Avoid using magic numbers or strings in the test cases.
2. Avoid Magic Numbers And Magic Strings
Using magic strings or numbers must be clarified for readers, making the tests less readable. In addition, it diverts readers from looking at the implementation details and makes them wonder why a particular value has been chosen instead of focusing on the actual test.
On the other hand, if a constant needs to be changed, changing it in one place updates all the different values. So, it is better to use variables or constants in the tests for assigning values. It would help you to express as much intent as possible while writing tests.
3. Write Deterministic Tests
Deterministic tests either pass all the time or fail all the time until fixed. But they exhibit the same behaviour every time they are run unless the code is changed. So a flaky test, also known as a non-deterministic test that sometimes passes and sometimes fails, is as good as having no difficulty.
To avoid non-deterministic tests, ensure they are completely isolated and are not dependent on other test cases. You can fix flaky tests by controlling external dependencies and environmental values like the machine’s current time or language settings.
4. Avoid Test Interdependencies
Test runners generally run multiple unit tests simultaneously without sticking to any particular order, so interdependencies between tests make them unstable and difficult to execute and debug. You should ensure each test case has its setup and teardown mechanism to avoid test interdependencies.
To write independent tests, do not assume anything based on the order in which you write test cases. If tests are coupled, isolate the code into small groups/classes to be tested independently. Otherwise, changes made to one test can affect other tests.
5. Test For Edge Cases
Edge cases are the scenarios that occur at the boundaries of the input data, and they are often the most critical to test. For example, in a test for a function that calculates the square root of a number, it is essential to test not only with positive numbers but also with negative numbers and zero. Edge cases can reveal bugs that may not be detected in routine testing, and they help ensure that the code is robust and can handle unexpected input.
6. Use Test-Driven Development (TDD)
Test-driven development (TDD) is a software development process where tests are written before the actual code. It follows the principle of “red, green, refactor,” where the developer first writes a test that fails (red), then writes the code that passes the test (green), and then refactors the code to improve its design. TDD encourages developers to think about the requirements and design of the legend before writing it, which leads to better-designed code that is more testable.
7. Use A Test Runner
A test runner is a tool that automates the execution of unit tests. It can run all tests at once, or it can run specific tests based on certain criteria. Test runners also provide helpful features like test result reporting, discovery, and filtering. Using a test runner can save time and effort, eliminating the need to run tests manually.
8. Keep Tests Simple
Tests should be simple and easy to understand. Avoid complex logic and nested loops in trials, as they can make it challenging to understand the test’s purpose and debug if the test fails. Also, tests should only cover one scenario and behaviour at a time.
9. Use Assert Libraries
Assert libraries provide a set of assertion functions that can be used to check the expected results of a test. They make it easy to express a trial’s desired outcome and identify the cause of a failure. Assert libraries also provide helpful error messages that help understand what went wrong when a test fails.
10. Automate Testing
Automating testing is essential for maintaining a high-quality codebase and ensuring that changes to the code do not introduce new bugs. Automating tests saves time and effort, eliminating the need to run tests manually.
In conclusion, Unit Testing is an essential part of the software development process, and it is important to follow best practices to ensure that tests are practical and efficient. Writing readable tests, avoiding magic numbers and strings, writing deterministic tests, avoiding test interdependencies, testing for edge cases, using TDD, using a test runner, keeping tests simple, using assert libraries, and automating testing, you can ensure that your code is robust and reliable.
Understanding Code Coverage

Code coverage measures how much of the code is executed by a test. It helps identify the untested parts of a codebase and can be used to indicate the quality of code. However, it is essential to note that code coverage is not the sole measure of code quality, but it can be a helpful tool.
Unit Testing can make it easier to achieve high code coverage since it breaks down the code into the smallest, reusable, and testable components. Automated unit tests can even help cover the entire codebase. However, achieving 100% code coverage might be difficult, so spending only a little effort is essential.
The goal for code coverage can vary depending on the project and team. A 100% code coverage might seem impossible initially, so even a goal of achieving 60-80% coverage is a good starting point. However, insufficient coverage numbers are a definite sign of weakness.
To increase code coverage, one can use parameterized tests and add more tests for more code paths or use cases of the method under test.
The Importance Of Unit Testing
Unit Testing is an essential aspect of software development and can be used to ensure that the code is robust and reliable. The above-mentioned best practices are not the only ways to maximize outcomes, but they can ease the Unit Testing process, with automation being the key.
Using frameworks available in various languages can simplify the process of Unit Testing. These frameworks provide advanced features that can be hand-coded for complex requirements. Unit Testing is focused and unique, and its outcome cannot be matched with any other type of testing.
Manish Surapaneni
A Visionary & Expert in enhancing customer experience design, build solutions, modernize applications and leverage technology with Data Analytics to create real value.
Other articles

Manish Surapaneni
Stop Outsourcing Your Ethics, Tech Leaders
Setting up their own ethics committee is a step forward for tech companies, as they are the clear winners of
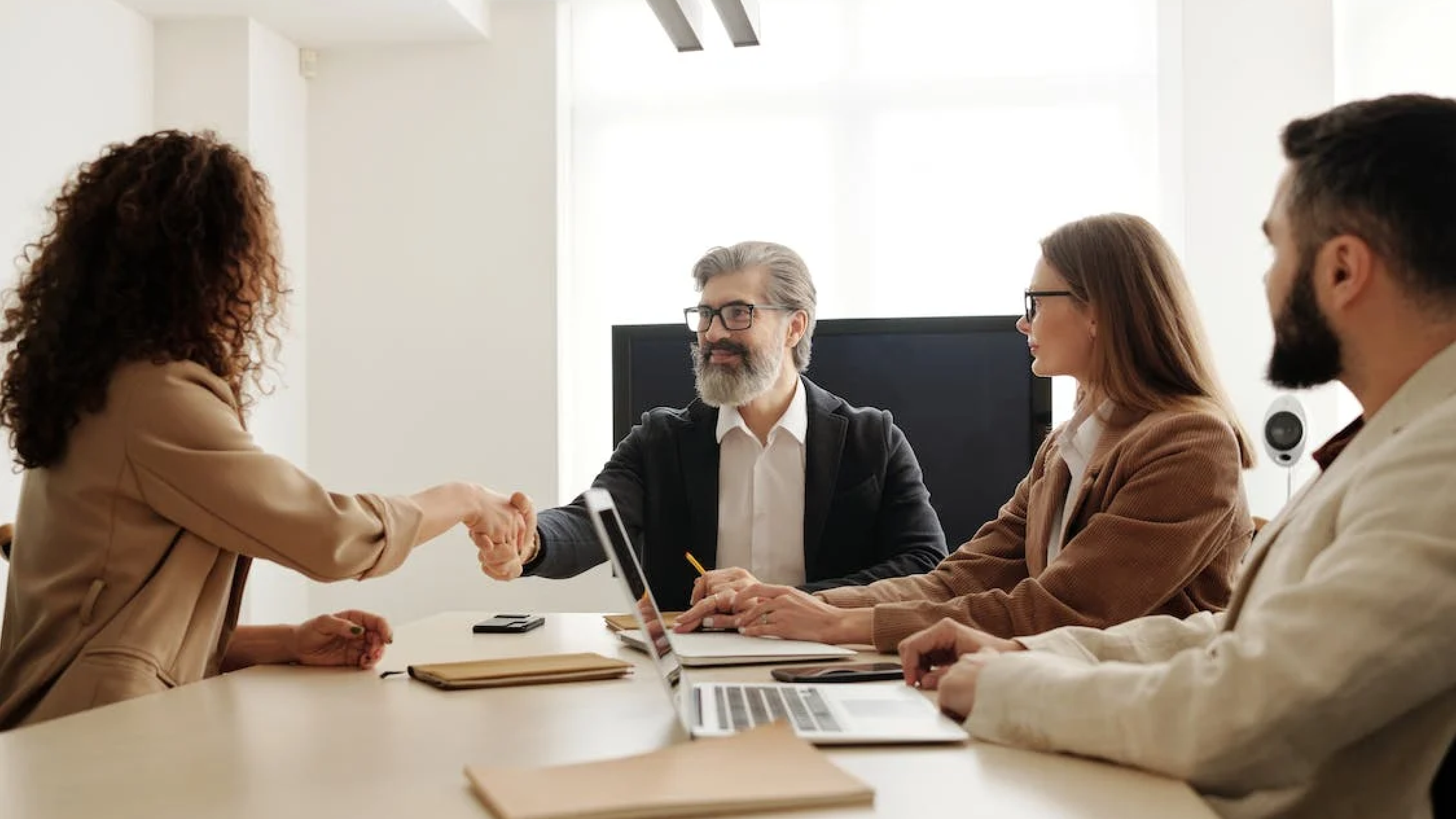
Manish Surapaneni
How To Beat The Tech Talent Squeeze In 2023: 6 Proven Strategies
In the current economic climate, with rising costs and increased job turnover, one of the biggest mistakes a company can

Manish Surapaneni
How Augmented Human Intelligence Can Enhance Our Lives With Machines
The advancement of technology has simplified our lives. It has also significantly increased our reliance on electronic devices. MIT Media